Symbolic Links
Apart from regular files, a file system may allow symbolic links to be created. A symbolic link (also called a hard link, a shortcut, or an alias) is a special file that acts as a reference to a directory entry, called the target of the symbolic link. Creating an alias of a directory entry in the file system is similar to creating a symbolic link. Using symbolic links is transparent to the user, as the file system takes care of using the target when a symbolic link is used in a file operation, with one caveat: Deleting the symbolic link does not delete the target. In many file operations, it is possible to indicate whether symbolic links should be followed or not.
21.2 Creating Path Objects
File operations that a Java program invokes are performed on a file system. Unless the program utilizes a specific file system constructed by the factory methods of the java.nio.file.FileSystems utility class, file operations are performed on a file system called the default file system that is accessible to the JVM. We can think of the default file system as the local file system. The default file system is platform specific and can be obtained as an instance of the java.nio.file.FileSystem abstract class by invoking the getDefault() factory method of the FileSystems utility class.
FileSystem dfs = FileSystems.getDefault(); // The default file system
File operations can access the default file system directly to perform their operations. The default file system can be queried for various file system properties.
static FileSystem getDefault()
Declared in
java.nio.file.FileSystems
Returns the platform-specific default file system. If the method is called several times, it returns the same FileSystem instance for the default file system.
abstract String getSeparator()
Declared in
java.nio.file.FileSystem
Returns the platform-specific name separator for name elements in a path, represented as a string.
Paths in the file system are programmatically represented by objects that implement the Path interface. The Path interface and various other classes provide factory methods that create Path objects (see below). A Path object is also platform specific.
A Path object implements the Iterable<Path> interface, meaning it is possible to traverse over its name elements from the first name element to the last. It is also immutable and therefore thread-safe.
In this section, we look at how to create Path objects. A Path object can be queried and manipulated in various ways, and may not represent an existing directory entry in the file system (p. 1294). A Path object can be used in file operations to access and manipulate the directory entry it denotes in the file system (p. 1304).
The methods below can be used to create Path objects. The methods are also shown in Figure 21.1, together with the relationship between the different classes of these methods. Note in particular the interoperability between the java.nio.file.Path interface that represents a path and the two classes java.io.File and java.net.URI. The class java.io.File represents a pathname in the standard I/O API and the class java.net.URI represents a Uniform Resource Identifier (URI) that identifies a resource (e.g., a file or a website).
static Path of(String first, String… more)
static Path of(URI uri)
These methods are declared in the java.nio.file.Path interface.
abstract Path getPath(String first, String… more)
This method is declared in the java.nio.file.FileSystem class.
static Path get(String first, String… more)
static Path get(URI uri)
These static methods are declared in the java.nio.file.Paths class.
Path toPath()
This method is declared in the java.io.File class.
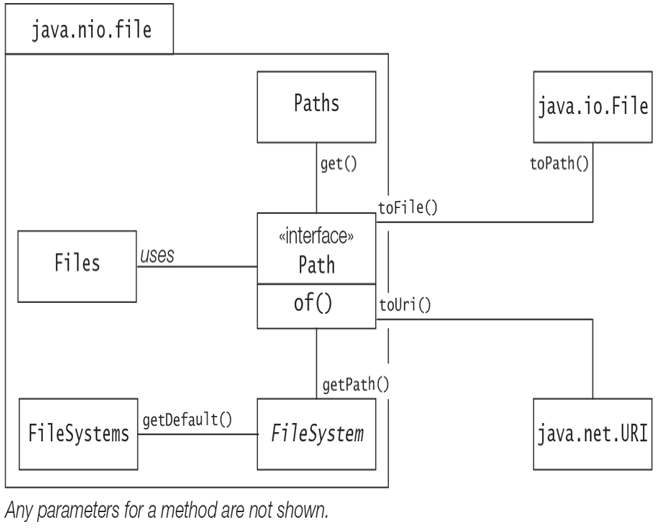
Figure 21.1 Creating Path Objects