Writing Binary Values to a File
To write the binary representation of Java primitive values to a binary file, the following procedure can be used, which is also depicted in Figure 20.2.
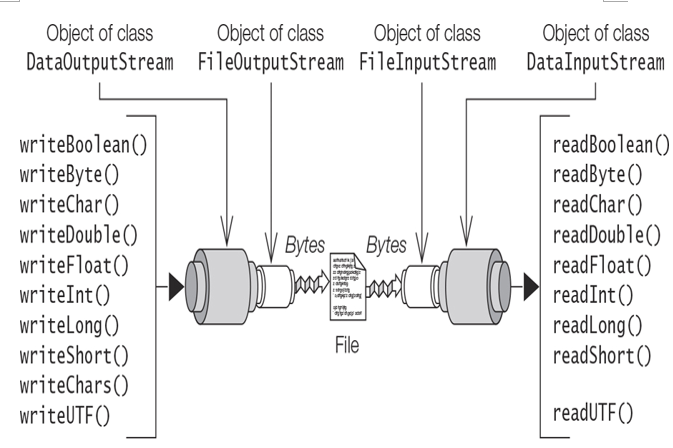
Figure 20.2 Stream Chaining for Reading and Writing Binary Values to a File
Use a try-with-resources statement for declaring and creating the necessary streams, which guarantees closing of the filter stream and any underlying stream.
Create a FileOutputStream:
FileOutputStream outputFile = new FileOutputStream(“primitives.data”);
Create a DataOutputStream which is chained to the FileOutputStream:
DataOutputStream outputStream = new DataOutputStream(outputFile);
Write Java primitive values using relevant writeX() methods:
Note that in the case of char, byte, and short data types, the int argument to the writeX() method is converted to the corresponding type, before it is written (see Table 20.3).
See also the numbered lines in Example 20.2 corresponding to the steps above.
Reading Binary Values from a File
To read the binary representation of Java primitive values from a binary file, the following procedure can be used, which is also depicted in Figure 20.2.
Use a try-with-resources statement for declaring and creating the necessary streams, which guarantees closing of the filter stream and any underlying stream.
Create a FileInputStream:
FileInputStream inputFile = new FileInputStream(“primitives.data”);
Create a DataInputStream which is chained to the FileInputStream:
DataInputStream inputStream = new DataInputStream(inputFile);
Read the (exact number of) Java primitive values in the same order they were written out to the file, using relevant readX() methods. Not doing so will unleash the wrath of the IOException.
See also the numbered lines in Example 20.2 corresponding to the steps above. Example 20.2 uses both procedures described above: first to write and then to read some Java primitive values to and from a file. It also checks to see if the end of the stream has been reached, signaled by an EOFException. The values are also written to the standard output stream.
Example 20.2 Reading and Writing Binary Values
import java.io.*;
public class BinaryValuesIO {
public static void main(String[] args) throws IOException {
// Write binary values to a file:
try( // (1)
// Create a FileOutputStream. (2)
FileOutputStream outputFile = new FileOutputStream(“primitives.data”);
// Create a DataOutputStream which is chained to the FileOutputStream.(3)
DataOutputStream outputStream = new DataOutputStream(outputFile)) {
// Write Java primitive values in binary representation: (4)
outputStream.writeBoolean(true);
outputStream.writeChar(‘A’); // int written as Unicode char
outputStream.writeByte(Byte.MAX_VALUE); // int written as 8-bits byte
outputStream.writeShort(Short.MIN_VALUE); // int written as 16-bits short
outputStream.writeInt(Integer.MAX_VALUE);
outputStream.writeLong(Long.MIN_VALUE);
outputStream.writeFloat(Float.MAX_VALUE);
outputStream.writeDouble(Math.PI);
}
// Read binary values from a file:
try ( // (1)
// Create a FileInputStream. (2)
FileInputStream inputFile = new FileInputStream(“primitives.data”);
// Create a DataInputStream which is chained to the FileInputStream. (3)
DataInputStream inputStream = new DataInputStream(inputFile)) {
// Read the binary representation of Java primitive values
// in the same order they were written out: (4)
System.out.println(inputStream.readBoolean());
System.out.println(inputStream.readChar());
System.out.println(inputStream.readByte());
System.out.println(inputStream.readShort());
System.out.println(inputStream.readInt());
System.out.println(inputStream.readLong());
System.out.println(inputStream.readFloat());
System.out.println(inputStream.readDouble());
// Check for end of stream:
int value = inputStream.readByte();
System.out.println(“More input: ” + value);
} catch (FileNotFoundException fnf) {
System.out.println(“File not found.”);
} catch (EOFException eof) {
System.out.println(“End of input stream.”);
}
}
}
Output from the program:
true
A
127
-32768
2147483647
-9223372036854775808
3.4028235E38
3.141592653589793
End of input stream.